This blog focuses on implementing Android’s in-app subscription purchase in React Native. Many steps are involved, making it confusing for beginners to follow a sequence of steps.
Step 1:
Add the following library in the android/app/build.gradle
implementation "com.android.billingclient:billing:6.0.1"
Now add the following line of code again to the same file but in the defaultConfig array/object
missingDimensionStrategy 'store', 'play'
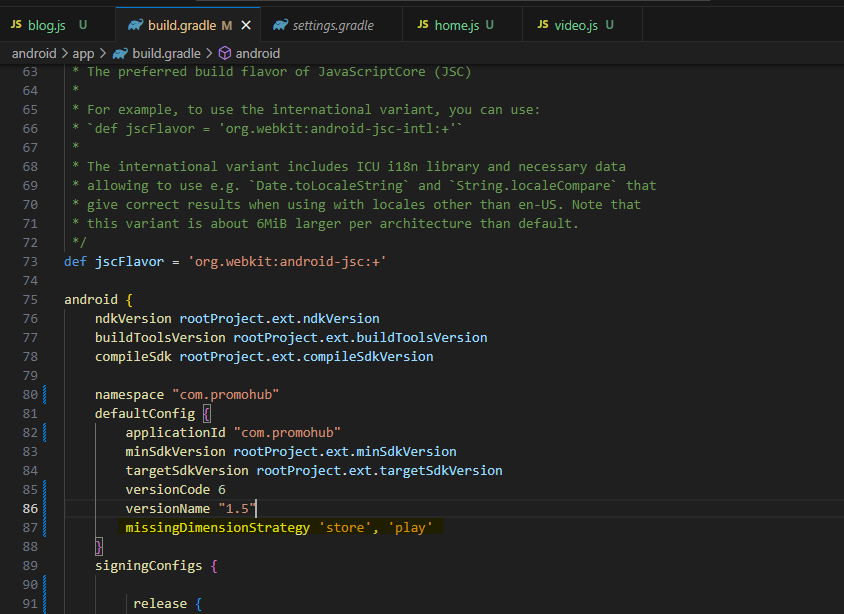
Step 2:
Build your app and create the aab file to upload to the Play Store. Once your app is uploaded, go to the products section of your application and select the subscription section.
If you have successfully implemented the above steps, you will see the option to create a new subscription. Follow the Play Store instructions to create the subscription.
Step 3:
You can use the following line of code to list all the subscriptions or products in your app in the form of JSON object.
import {initConnection, getSubscriptions,requestSubscription} from "react-native-iap"
Use this line to import the IAP Library
const params = Platform.select({
ios: { },
android: {
skus: ['your subscription ID']
}
})
The above code is used to set your SKUS. In the SKUS array, you must pass the ID of the product or subscription you created in the last step.
initConnection().then(async ()=>{
const subscriptions = await getSubscriptions(params);
});
The above code will initialize the connection to the IAP, and getSubscriptions function allows you to get the data of your subscriptions created on play store.
async function handlePurchase() {
getSubscriptions(params)
.then((value) => {
requestSubscription({
subscriptionOffers: [
{
sku: "your subscription ID",
offerToken:
value[0]["subscriptionOfferDetails"][0]["offerToken"],
},
],
})
.then((value) => console.log(value))
.catch((error) => console.log(error));
})
.catch((err) => console.log(err));
}
This handlePurchase() function can be called on button click and it will initiate the purchase process of subscription.
const checkSubscriptionStatus = async () => {
try {
const purchases = await getAvailablePurchases();
const activeSubscriptions = purchases.filter(
(purchase) => purchase.productId === 'your subscription ID' && purchase.transactionReceipt
);
if (activeSubscriptions.length > 0) {
// User has an active subscription
const expirationDate = new Date(activeSubscriptions[0].transactionReceipt.purchaseTime);
setSubscriptionStatus("active");
} else {
// User doesn't have an active subscription
setSubscriptionStatus("inactive");
}
} catch (error) {
// Handle error
}
};
checkSubscriptionStatus() function will help you identify the status of the subscription of the users.